Microfrontend Component
The Microfrontend
component provides a versatile way to compose modular user interface elements by enabling the integration of one micro frontend into another. With this component, developers are able to specify which micro frontend to include, where in the parent micro frontend it should be mounted, and how to provide extra options to modify its behavior.
Basic usage example
Importing and using the component
import { Microfrontend } from '@resultadosdigitais/front-hub/react'
const YourComponent = () => {
return (
<>
...other_code
<Microfrontend name={MFE_NAME} {...rest} />
...other_code
</>
)
}
export default YourComponent
And register the version to be requested in the front-hub.config.js
.
...rest_of_configs,
mfeDependencies: ['MFE_NAME@latest']
If you are requesting another team micro frontend, we recommend thinking about using a fixed version and manually updating when needed. Click here to know more
Properties
name
The micro frontend name as specified in the package.json without the npm scope @.../
.
<Microfrontend name="mfe-name" />
Fixed version (name+version)
As shown in the example above, not specifying a version and with @latest
in the mfeDependencies
, will fetch the latest active version from Fronthub. If you need to require a fixed version, for any reason or if you are requesting multiple versions of the same micro frontend in one project, you can do so as follows.
<Microfrontend name={MFE_NAME@THE_SEMVER_VERSION} />
What is THE_SEMVER_VERSION
priority
This property has two options, high
and medium
. It determines the priority with which FrontHub will request the micro frontend assets. When the option is medium the micro frontend will be loaded after the property document.readyState
be complete
. This feature is especially useful to delay the request of non-essential micro frontends, thereby improving overall page performance. The default option is high
.
<Microfrontend name="mfe-name" priority="medium" />
errorCallback
A function to handle any errors that occurs during the micro frontend require process.
<Microfrontend
name={MFE_NAME}
erroCallback={error => {
MyCustomErrorService.error(error)
console.error(`Failed to load MFE: ${error}`)
}}
/>
renderWrapper
Function responsible for rendering a wrapper around the micro frontend that is being requested. It provides a children
that is the micro frontend to be rendered
<Microfrontend
name={MFE_NAME}
renderWrapper={({ children }) => (
<YourCustomWrapper>{children}</YourCustomWrapper>
)}
/>
fallback
A ui component to render while the micro frontend is being requested and is not yet rendered in the screen.
<Microfrontend name="mfe-name" fallback={<LoadingComponent />} />
props
Object that allows you to pass on additional information to the micro frontend context. The provided object must be plain, without any functions or callbacks. If it is needed to use functions or callbacks, please refer the communication documentation for further guidance.
The information passed within the object should only include data that is not already present in the Fronthub Context, as this object will be merged with it. Overwriting existing context data may lead to unintended behavior.
Click here to know how to access these props in the micro frontend.
const obj = {
name: 'foo'
age: 18
}
<Microfrontend name="mfe-name" props={obj}/>
…rest
Information that will be passed down to the HTML element that the micro frontend will be rendered on.
MFE Dependencies
This key located in front-hub.config.js
functions similarly to project a dependency, in case your micro frontend doesn't have one, make sure to update Fronthub to the last version and manually add this key to the end of the file. Specifying the micro frontend version in the front-hub.config.js
file helps prevent potential disruptions to the application and aids developers to understand which micro frontend that project depends on.
It's strongly recommended to use a fixed version, especially when requesting a micro frontend developed by another team. This precaution is essential because changes made by external teams might inadvertently impact your application differently from theirs.
In the file front-hub.config.js
, you'll find a key named mfeDependencies
, which is designated for specifying the versions of micro frontends that will be required.
...rest_of_configs,
mfeDependencies: ['MFE_NAME@SEM_VER_VERSION', 'OTHER_MFE_NAME@latest']
How to version it
There are two possible way of version it
Fixed version
Specify the SEM_VER_VERSION version that you need after the name. With this way, it will be needed to manually update the micro frontend.
...rest_of_configs,
mfeDependencies: ['MFE_NAME@SEM_VER_VERSION']
In a real world would loke like
...rest_of_configs,
mfeDependencies: ['mfe-dashboard@1.0.4']
And to request in the code
<Microfrontend name="mfe-dashboard@1.0.4" props={obj} />
Latest active version
Add @latest
after the name. This will require the latest active version in Fronthub for that micro frontend and is not needed to manually update any version. Remembering that due the rollback mechanism, the active version may not be the last one deployed. This one work the same way of nothing fixing a version in the requireMicrofrontend.
...rest_of_configs,
mfeDependencies: ['OTHER_MFE_NAME@latest']
In a real world would loke like
...rest_of_configs,
mfeDependencies: ['mfe-dashboard@latest']
And to request in the code
<Microfrontend name="mfe-dashboard" props={obj} />
Local development
To help the development with this component, it's possible to render the micro frontend that is being required from staging or using the Fake repository feature without the need to deploy everything in staging.
Rendering from Staging
In the front-hub.config.js
file, add at the beginning of the object the key with the value:
repository: 'https://THE_FRONT-HUB_STAGING-URL',
...rest_of_configs,
If you require assistance in determining the URL, please contact the Front-hub team for support.
From Fake repository
It is possible to run two micro frontends both in the local development. For that it is needed to run the fake repository in the micro frontend that will be requested. And, in the micro frontend that is requesting, add in the front-hub.config.js
the config below. Remember to restart the application
repository: 'http://localhost:3001', // or another port that fake repository returned
...rest_of_configs,
Use cases
Experiments
A compelling use case for this component is to decouple logic and expedite experimentation. Instead of coding directly within other team's projects, developers can build the entire application externally and utilize this component to seamlessly integrate the experiments.
In the image below for example, this entire sticky bar in black can be another micro frontend called by the navbar and is developed by a different team in another repository.

Detach and re use
A fundamental concept of micro frontends is to create smaller, modular applications, akin to building with LEGO bricks. This approach allows for the construction of applications where components can be reused across multiple pages. Consider the following example:
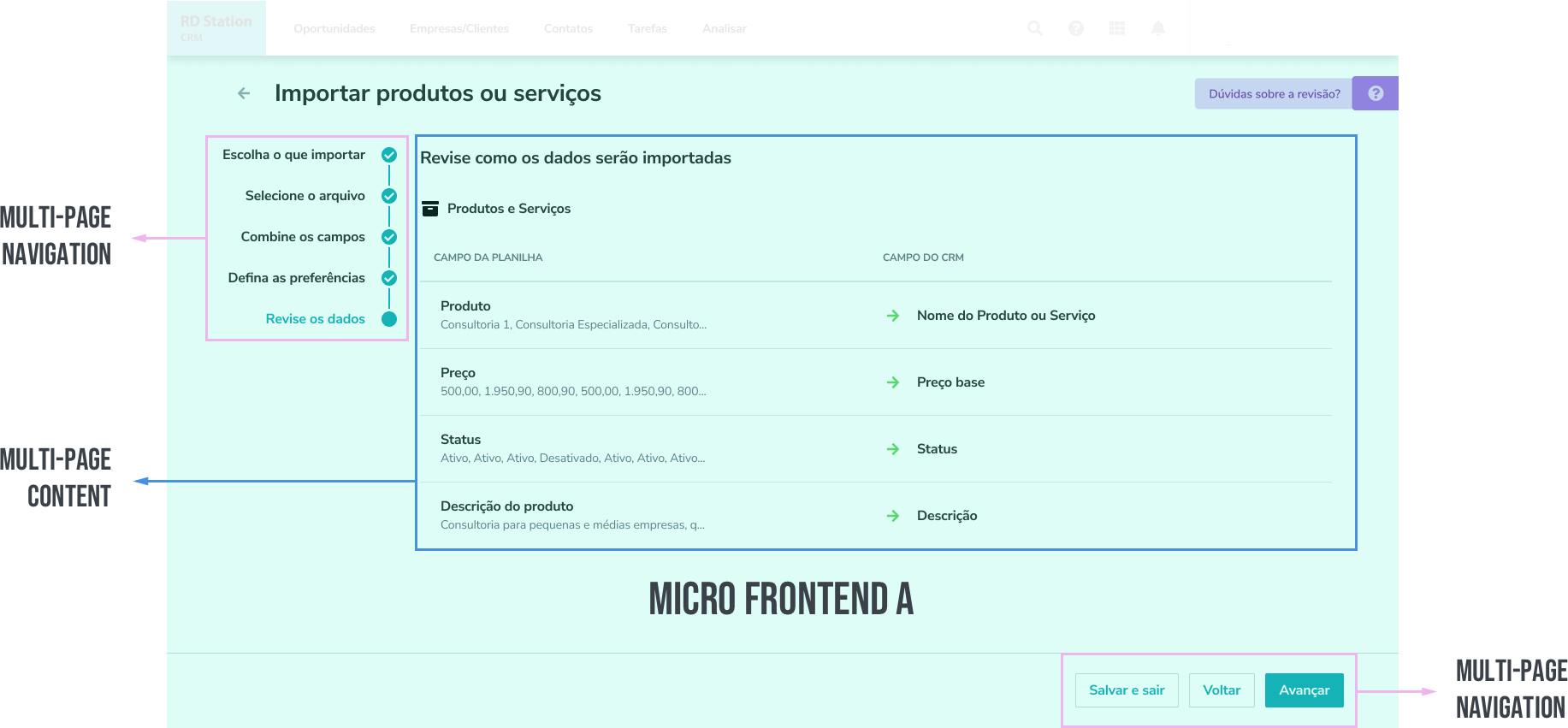
Widgets
One common capability enabled by this component is the creation of micro frontend widgets. These are small applications that typically operate independently and can be seamlessly integrated into any page or micro frontend. Examples include support chat widgets, shopping cart components for marketplaces, and more.
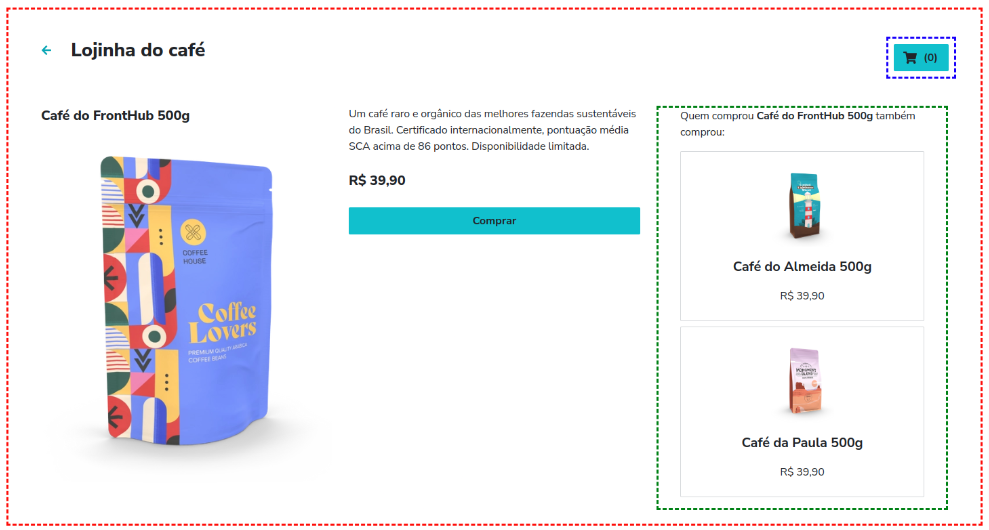
Testing
This component does not support integration tests directly. The intention is not to request and render the micro frontend within unit tests. However, to simplify testing, developers can utilize a custom selector called getMicrofrontendByName
.
It is possible to use this selector in two different ways
import {
renderWithFrontHub,
getMicrofrontendByName,
} from '@resultadosdigitais/front-hub/react/jest'
const { getMicrofrontendByName } = renderWithFrontHub(<YourComponent />)
And to select the micro frontend
it('renders with mfe correctly', async () => {
const { getMicrofrontendByName } = renderWithFrontHub(<YourComponent />)
const mfe = getMicrofrontendByName('the_microfrontend_name')
expect(mfe).toBeInTheDocument()
expect(mfe.props).toEqual({ your: 'prop' })
expect(mfe.priority).toEqual('medium')
})
Examples
How to request the latest
In the front-hub.config.js
mfeDependencies: ['sidebar@latest']
In the code
<Microfrontend name="sidebar" />
How to request with a fixed version
In the front-hub.config.js
mfeDependencies: ['sidebar@1.0.1']
In the code
<Microfrontend name="sidebar@1.0.1" />
How to request multiple micro frontends
In the front-hub.config.js
mfeDependencies: ['navbar@1.0.1', 'navbar@1.0.2', 'sidebar@latest']
In the code
/// With fixed version
<Microfrontend name="navbar@1.0.1" />
/// As latest
<Microfrontend name="sidebar" />
How to request the same micro frontend multiple times
In the front-hub.config.js
mfeDependencies: ['button@latest', 'button@1.0.1', 'button@1.0.2']
In the code
/// The one that will request the latest active version
<Microfrontend name="button" />
/// Any additional should use a fixed version that cannot be duplicated.
<Microfrontend name="button@1.0.1" />
<Microfrontend name="button@1.0.2" />
Troubleshooting
Log Errors
A guideline to assist in debugging errors related to versioning requests:
Error | Reasons | Solution |
---|---|---|
MFE mfeName not found with a valid version. | - The micro frontend that you are requesting is not registered in mfeDependencies . - A registered micro frontend in mfeDependencies does not have a version @latest . | In the front-hub.config.js , ensure that the micro frontend you are calling is registered under mfeDependencies . If you are calling it multiple times, verify that you are passing the correct registered version each time. |
Requested MFE mfeName is different from the found mfeDependencies | - The micro frontend version that you are requesting is not the one registered in mfeDependencies . | Verify whether the version specified in the component matches the one registered in the front-hub.config.js file. |
Found multiple dependencies for MFE mfeName , identify which version from [...] | - There are multiple micro frontend versions registered in mfeDependencies , none of them is latest, and you are not specifying the version in the component. | If you are not specifying a version for the micro frontend in the component, ensure to include one of the versions registered in the front-hub.config.js file, or register a new version with @latest as the version. |
In the Code
MFE mfeName not found with a valid version.
Missing the micro frontend register
In the front-hub.config.js
mfeDependencies: []
In the code
<Microfrontend name="sidebar@1.0.1" />
Calling multiple micro frontends
In the front-hub.config.js
mfeDependencies: ['sidebar@1.0.1', 'sidebar@1.0.2']
In the code
<Microfrontend name="sidebar@1.0.1" />
<Microfrontend name="sidebar@1.0.3" />
Requested MFE mfeName is different from the found mfeDependencies
In the front-hub.config.js
mfeDependencies: ['sidebar@1.0.1']
In the code
<Microfrontend name="sidebar@1.0.3" />
Found multiple dependencies for MFE mfeName, identify which version from [...]
In the front-hub.config.js
mfeDependencies: ['sidebar@1.0.1', 'sidebar@1.0.2', 'sidebar@1.0.3']
In the code
<Microfrontend name="sidebar" />